继承
# 继承
# 概念
类型和类型之间的关系
# 目的
把子类中的成员提取到父类中,实现代码重用
# 方式
- 原型继承
function Person (name, age) {
this.name = name;
this.age = age;
this.eat = function () {
console.log('吃饭');
}
}
Person.prototype.sleep = function () {
console.log('睡觉');
}
function Child (sex) {
this.sex = sex;
}
// 将原型对象设为父类的实例
Child.prototype = new Person();
// 将构造器指向自身的构造函数
Child.prototype.constructor = Child;
var c = new Child('男');
var p = new Person('人类总的', 10);
console.dir(c);
console.dir(p);
console.log(c instanceof Child);
console.log(c instanceof Person);
console.log(c instanceof Object);
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
- 输出:
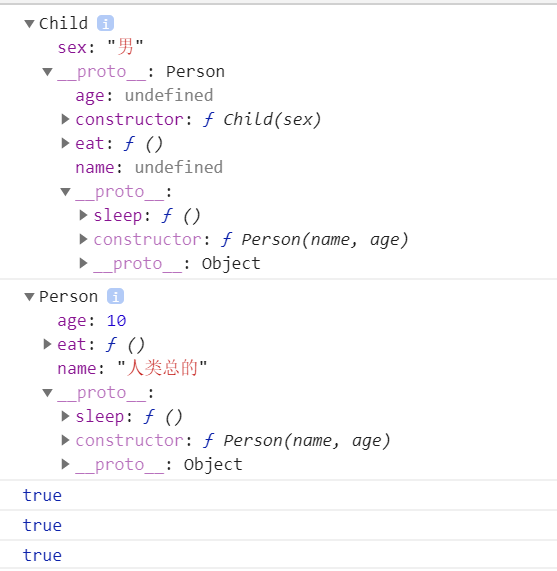
- 缺点:
- 无法设置父类属性的值
- 注意:
- 子类实例对象的原型对象的原型对象 === 父类构造函数的原型对象,所以
子类实例对象 instanceof 父类 === true
- 子类实例对象的原型对象的原型对象 === 父类构造函数的原型对象,所以
借用构造函数
function Person(name, age) { this.name = name; this.age = age; this.eat = function () { console.log('吃饭'); } } Person.prototype.sleep = function () { console.log('睡觉'); } // name和age是父类的属性,sex是子类的属性 function Child(name, age, sex) { // 借用构造函数,使用call方法 Person.call(this, name, age); this.sex = sex; } var c = new Child('我是子类', 18, '男'); var p = new Person('我是父类', 10); console.dir(c); console.dir(p); console.log(c instanceof Child); console.log(c instanceof Person); console.log(c instanceof Object);
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23输出:
- 缺点:
- 父类的原型对象的属性和方法无法继承
- 注意:
- 父类的非原型对象的属性和方法会直接添加到子类的实例对象上
- 子类实例对象的原型对象的原型对象 === Object的原型对象,非父类的原型对象,即子类与父类无原型链上的关联,所以
子类实例对象 instanceof 父类 === false
组合继承 (借用构造函数继承 + 原型继承)
function Person(name, age) { this.name = name; this.age = age; this.eat = function () { console.log('吃饭'); } } Person.prototype.sleep = function () { console.log('睡觉'); } function Child(name, age, sex) { // 借用构造函数继承 Person.call(this, name, age); this.sex = sex; } // 原型继承,不要等于Person.prototype Child.prototype = new Person(); // 添加构造器 Child.prototype.constructor = Child; // 子类的原型对象私有的方法 Child.prototype.drinknainai = function () { console.log('喝奶'); } var c = new Child('我是子类', 18, '男'); var p = new Person('我是父类', 10); console.dir(c); console.dir(p); console.log(c instanceof Child); console.log(c instanceof Person); console.log(c instanceof Object);
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
输出:
注意:
- 在使用原型继承的时候,不用使用
子类.prototype = 父类.prototypr
,如Child.prototype = Person.prototype
,因为会和父类的原型对象共用一个地址,导致子类无法在自己的原型对象上添加自己私有的属性和方法,一旦添加,父类的原型对象也会改变,导致混乱
- 在使用原型继承的时候,不用使用
上次更新: 2020/12/06, 21:12:00